Quick tutorial JavaScript with D3.js
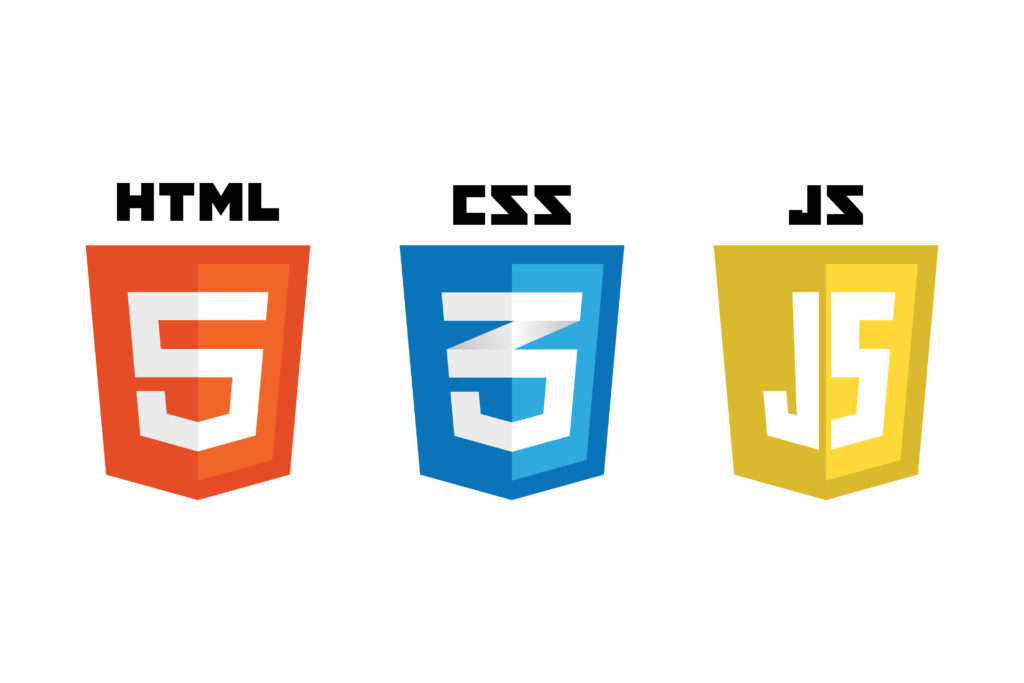
Introduction
About this passage
This passage simply introduces how to combine HTML, CSS and Javascript together to design a web page. Then D3.js, a javascript package for data visualization is introduced and applied to visualize some simple data.
About HTML, CSS, JavaScript
HTML: HTML stands for Hyper Text Markup Language. It is the standard markup language for Web pages. Elements in HTML are the building blocks of HTML pages, represented by <> tags
CSS: it stands for Cascading Style Sheets. It describes how HTML elements are to be displayed.
JavaScript: JavaScript is the Programming Language for the Web. It can update and change both HTML and CSS. It can calculate, manipulate and validate data
Combining HTML, CSS, JavaScript for a webpage
To start with, Let’s create a folder that will contain all HTML, CSS, JavaScript files we will create.
Write a HTML file
First, we write an empty HTML file with title “Demo” and empty content for passage <p> <\p> and heading <h1><\h1>
1 | <html> |
Then we put Javascript into HTML directly
1 | <html> |
The tag <\script><\script> tells HTML the code inside this block is Javascript. In this script, we select the element with tag name ‘h1’ and ‘p’ using document.getElementsByTagName() and then input text “Hello I’m Header” and “I’m Passage”. This will let HTML display such texts in corresponding elements.
Using Javascript file instead of internal script
To use JavaScript file rather than script inside HTML, we first create a JavaScript file called “myjs.js” in the same folder. Then copy the JavaScript code from HTML to Javascirpt.
1
2
3
4
5
6
7
8
9
10var headers = document.getElementsByTagName("h1");
for (var i = 0; i < headers.length; i++) {
var header = headers.item(i);
header.innerHTML = "Hello I'm Header";
}
var paragraphs = document.getElementsByTagName("p");
for (var i = 0; i < paragraphs.length; i++) {
var paragraph = paragraphs.item(i);
paragraph.innerHTML = "I'm Passage";
}
In HTML file, we replace the script with a sentence, shown as below.
1 | <html> |
“id”,”type” attributes tell HTML we are looking for a file with name “myjs” and file type of JavaScript.
Define style with CSS file
- Internal CSS code
Elements’ styles, like color, font size, etc can be defined using CSS code. To add CSS inside HTML, here is an example:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44<html>
<head>
<meta charset="utf-8">
<title>D3 Demo</title>
</head>
<style>
h2 {
color: blue;
font-size: 12px;
font-family: "Open Sans";
text-anchor: middle;
}
</style>
<body>
<p>Hello World </p>
<h1></h1>
<script id="myjs" src="myjs.js" type="text/javascript"></script>
</body>
</html>
~~~
The tag \<style> tells the HTML the style settings (blue color, font-size = 12 pixel, ...) for all h2 element.
<br>
- __External CSS code__
Usually, using a separated css file, rather than internal css code inside HTML file, can help further improvement for our project. It is more flexible to use css for element style design.
To use external CSS file, replace the style codes in HTML with \<link> tag
~~~HTML
<html>
<head>
<meta charset="utf-8">
<title>D3 Demo</title>
</head>
<link id ="mystyle" href="mystyle.css" rel="stylesheet" type="text/css" />
<body>
<p>Hello World </p>
<h1></h1>
<script id="myjs" src="myjs.js" type="text/javascript"></script>
</body>
</html>
Then Create a CSS file, called “mystyle.css” and copy the style code to CSS file
1 | h2 { |
The CSS file is linked to the HTML file by <link> tags in HTML. “id” is the identity of element “link” and “href” is set to be “mystyle.css” file. Attribute “type” is the file type of “mycss.css” file, hence it is css or text type.
What is D3.js
D3.js is a JavaScript library for manipulating documents based on data. D3 helps you bring data to life using HTML, SVG, and CSS. D3’s emphasis on web standards gives you the full capabilities of modern browsers without tying yourself to a proprietary framework, combining powerful visualization components and a data-driven approach to DOM manipulation
Import D3.js
To import D3.js library, we can either add this line to the HTML file, then the D3.js library from the website will be applied.
1 | <script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script> |
Or download D3.js library from https://d3js.org/ to the folder directly.
Here is an example of importing D3.js library and my javascript (D3-demo.js), css files (mystyle.css)
1 | <html> |
Selection, Insertion, Remove for elements
- Selection and Modification
1 | // Using JavaScript to change URL to stylesheet and JavaScript in HTML |
- Bar Plot
1 | // Setup Canvas element by appending tag <svg> into <body> section |
Copy all codes above and open our HTML file in browser, we can see something like this.
Reference
[1] https://wiki.jikexueyuan.com/project/d3wiki/selection.html
[2] https://d3js.org/
[3] https://www.w3schools.com/