Java-2-BasicGrammar
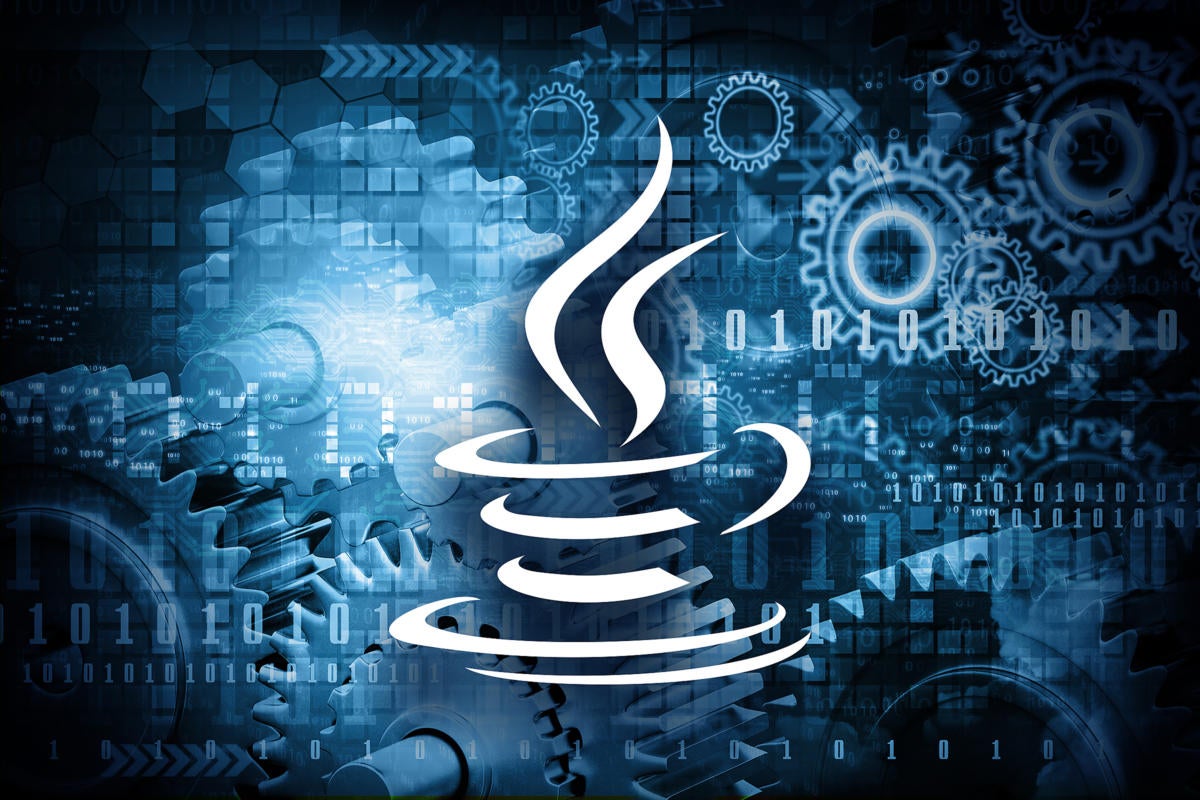
1. Introduction
这篇文章主要介绍Java的基本语法: 数据类型, 注释类型, 泛型(Generic Type, 等同于C++的Template)。之后再简单写个Java Demo去跑一遍程序
2. Data Type
整型(byte、short、int、long)
整型数据有四种,它们的取值范围不同
byte 的取值范围:-128~127(-2的7次方到2的7次方-1)
short 的取值范围:-32768~32767(-2的15次方到2的15次方-1)
int 的取值范围:-2147483648~2147483647(-2的31次方到2的31次方-1)
long 的取值范围:-9223372036854774808~9223372036854774807(-2的63次方到2的63次方-1)
2.1 浮点型
浮点型包括float和double两种,区别在与精度,float是单精度、32位、符合IEEE 754标准的浮点数;double 数据类型是双精度、64 位、符合 IEEE 754 标准的浮点数。
float(单精度浮点型)取值范围:3.402823e+38~1.401298e-45
double(双精度浮点型)取值范围:1.797693e+308~4.9000000e-324
Note: java里面的浮点数,比如2.4, java默认会使用double。所以如果像 float a = 1.5 这样Java会自动把 a先设成double然后强制转换为float,这样编译时会报错。
2.2 字符类型
char 类型是一个单一的 16 位 Unicode 字符;
最小值是 \u0000(十进制等效值为 0);
最大值是 \uffff(即为 65535);
char 数据类型可以储存任何字符;
2.3 布尔类型
布尔类型只有两个取值,分别是true 、false 。
java默认是false。
2.4 Array
Java 的Array数组类型使用方法类似C/C++的array, 不过创建时要用new keyword
1 | //Indicate an array |
Notes:
array must be initialized by keyword new and size of array must be provided. Or we can directly initialize array by setting element values
1
2
3
4
5
6
7int[] arr = {1,2,3,4,5};
//or
int[] arr = new arr[5];
int[] arr = {1,2,3,4,5};
int[] arr;
arr= {1,2,3,4,5}; //error在初始化后, array的size不能更改
3. Variable 变量
3.1 类 变量
Java里面的变量分为:
local variable局部变量:
变量只能在作用域{..}或方法里面使用,在方法使用后,变量内存会被释放销毁,局部变量在使用时必须进行赋值操作或被初始化,否则出现编译错误。 例如1
2
3
4
5
6public class Cat{
public void print(){
String name = "cat"; // local variable, it can be used only inside method print()
System.out.println(name);
}
}类静态变量
静态变量在类中以 static 关键字声明,但必须在方法之外。无论一个类创建了多少个对象,类只拥有类变量的一份拷贝。生命周期与类一致。类的静态变量用于描述类的信息而不是实例的信息。instance variable实例变量
实例变量也叫类成员变量,声明在一个类当中,方法、构造方法和语句块之外。实例变量的生命周期与对象一致。
- constant常量
初始化之后数据就不能变动的变量,因此叫做常量。一般用final修饰的variable都是常量1
2
3public class Cat{
final String name="Mike";
}
3.1 类型变换
数据处理时常会要把一个数据类型转换成另外一种类型。而通常把占用字节空间大的类型变成占用字节少的类型时会导致数据精度的丢失,下面这幅图展示了类型变换时的精度丢失情况。
3. Annotation注释
注解,一种元数据形式,提供有关程序的数据,该数据不属于程序本身。注释对其注释的代码的操作没有直接影响。
注释的作用有:
- 编译前:为编译器提供编译检查的依据,辅助检查代码错误或抑制检查异常。
- 编译中或发布时:给编译器提供信息生成代码或给其他工具提供信息生成文档等。
- 运行时:在运行过程中提供信息给解释器,辅助程序执行。
- 注解经常和反射结合起来用,多用于框架中。
4. Generic Type泛型
在介绍 Generic 泛型前先来考虑一下这个问题, 在Java的ArrayList Class里面
1 | public class ArrayList { |
如果我们用这个ArrayList 去存放 String类型数据, 就必须强制转换Object到String类型,比如
1 | ArrayList list = new ArrayList(); |
这样的缺点有:
- 需要强制转换
- 不方便,容易类型转换不当而报错
为了解决这个问题, 其中一个解决方法就是把ArrayList里面的Object类型在每次使用前都变成我们所需的储存类型(如String, Integer,….). 而最好的方法就是ArrayList除了数据类型外,其他的所有variable, method都不变。这个时候就需要用到泛型Generic.
泛型的本质是为了参数化类型(在不创建新的类型的情况下,通过泛型指定的不同类型来控制形参具体限制的类型)。也就是说在泛型使用过程中,操作的数据类型被指定为一个参数,这种参数类型可以用在类、接口和方法中,分别被称为泛型类、泛型接口、泛型方法.
例子:
1 | // 创建ArrayList<Integer>类型: |
创建一个Generic Type Class:
1 | class House<T>{ |
Note: 泛型Class里面的array数组不能直接初始化,因为还不知道T的类型,所以要先通过用Object创建数据类型,然后强制转换为 (T[])数组类型
5. Coding Practice
1 | /* Java Review Task 2: DataType |
编译一下java文件
1 | javac DataType.java #use java compiler to compile java source code to byte code |
输出结果:
1 | Testing datatype... |
6. Conclusion
总结一下Java相对于C/C++, Python要注意的地方:
- 凡是像 3.14,这样的带小数点数字,java默认为double类型,如果要把它变成float,就要
float a= 3.14f
后面加个f. - Java 创建数组时,要么像C/C++那样直接
int array[];
或者int[] array
申明,要么直接int[] array ={1,2,3...}
来申明并初始化。还有一种是int[] array = new int[100]
这样来初始化但不赋值。Java里面一般是用int[] array
把type和[]一起写的写法 - C/C++ 初始化数组时是
int arr[]= {1,2,3....}
或者int arr[100]
- Python 初始化数组是直接
arr=[]
,arr=[0]*n
之类的 - Generic Type泛型里面一般不直接用T 类型 来初始化数组因为T 类型是未定的,但可以用Object类型再强制转换或者其他方式初始化
- Java里面的System.out.println()是把input当成字符串那样直接通过”+”加号把多个输入串起来, 而printf()是和C的printf一样要输入格式如%d之类的。
6. Reference
[1] Datawhale: https://github.com/datawhalechina/team-learning-program/blob/master/Java/11.%E6%B3%9B%E5%9E%8B.md
[2] Java Tutorial: https://www.liaoxuefeng.com/wiki/1252599548343744/1265102638843296